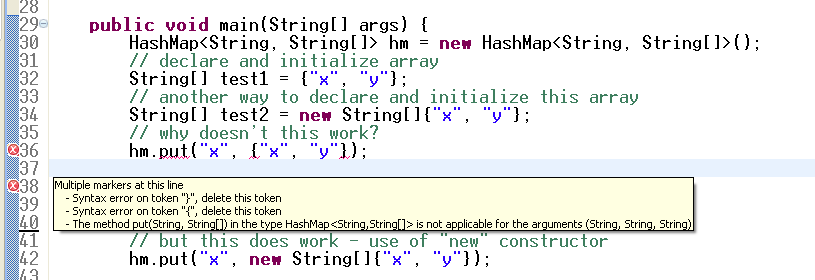
array initialization in java Originally uploaded by rocannon1980
Yesterday I had a little adventure in coding when trying to initialize and declare a Java array "on the fly", so to speak. The problem is illustrated by the screenshot; here's the code:
public class TestMe {
public static void main(String[] args) {
HashMap<String, String[]> hm = new HashMap<String, String[]>();
// Declare and initialize array. This is legal.
String[] test1 = {"x", "y"};
// Another way to declare and initialize this array, also legal.
String[] test2 = new String[]{"x", "y"};
// why doesn't this work??? Causes syntax error.
hm.put("x", {"x", "y"});
// This *does* work - use of "new" operator
hm.put("x", new String[]{"x", "y"});
}
}
The line which produces a syntax error in this code is
hm.put("x", {"x", "y"});
. The syntax error reads:Multiple markers at this line
- Syntax error on token "}", delete this token
- Syntax error on token "{", delete this token
- The method put(String, String[]) in the type HashMap is not applicable for the arguments (String, String, String)
Here, the compiler did not take the use of curly braces to be the shortcut syntax for declaring and an initializing the array.
I had started out by using the shortcut syntax, and when I saw the compiler error I thought I was losing it. It took some stumbling around to figure out that I could use the
new
operator to get the compiler to understand what I meant. Strange!I can't imagine this kind of question would ever come up on an interview, but you never know. I hope that I'll remember it from now on; it's the kind of thing that's easy to forget.
No comments:
Post a Comment